Feb 14, 2023
Publish Your Own NPM Package in 2 Minutes
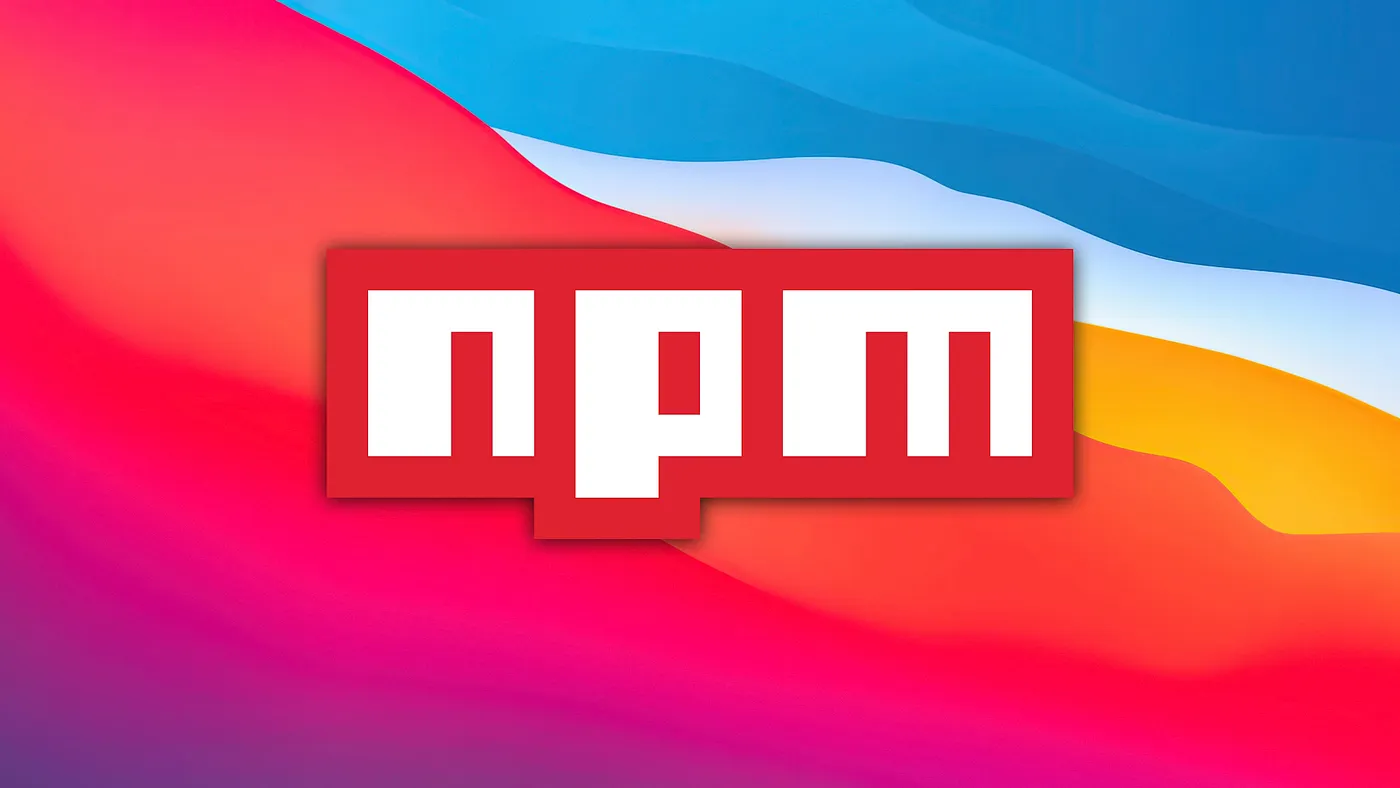
In this article, you'll learn how to publish your own npm package on the public npm registry in under 2 minutes.
Set up your npm account
Create an npm account
Before you can publish a package on the public npm registry, you'll need to create a new npm account by visiting: https://www.npmjs.com/signup.
Log into your account
Once you've done that, you'll need to log into your account using the npm login
command:
$ npm login
Which will generate an .npmrc
file in your home directory containing your credentials.
Note that you can display the npm username of the currently logged-in user using:
$ npm whoami
Write a simple Node.js module
Now that you're logged in, let’s create a new directory named hello-world
:
$ mkdir hello-world
$ cd hello-world
And within it, a new file named index.js
:
$ touch index.js
That exports the following helloWorld()
function:
function helloWorld() {
console.log('Hello World');
}
module.exports = helloWorld;
Unscoped vs scoped packages
Unscoped packages (default)
An unscoped package is a package that is always public — which means downloadable by everyone — and directly referred to by its name.
$ npm install --save hello-world
Its name has to be unique across the public registry.
Scoped packages
A scoped package is a package that is prefixed with a user or an organization name, and that can be either public — which means downloadable by everyone — or private — which means only downloadable by you or members of your organization.
$ npm install --save @username/hello-world
Since the user or the organization name acts as a form of namespace, its name doesn’t have to be unique across the public registry.
Initialize the module
Initializing a module essentially consists in creating its package.json
file, which is a manifest that includes the list of packages it depends on, as well as its name, description, author, license, and so on.
To initialize an unscoped module, you can use the npm init
command:
$ npm init
Which will prompt you with a list of questions you can answer by either pressing ENTER
to keep the default value, or typing your own value followed by ENTER
.
When prompted with this final question, press ENTER
once again to generate the package.json
file.
{
"name": "@username/hello-world",
"version": "1.0.0",
"description": "A hello world console program",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [
"hello"
],
"author": "John Doe",
"license": "MIT"
}
Is this OK? (yes)
Note that to initialize a scoped module, you can use the same command with the --scope
flag:
$ npm init --scope=@username
Publish your package
To publish an unscoped package on the public npm registry, you can use the npm publish
command:
$ npm publish
To publish a scoped package, you can use the same command alongside with the --access
flag to specify its visibility:
$ npm publish --access public
Upon completion, you should see the following log in your terminal window confirming that your package has been successfully published to the npm registry:
npm notice Publishing to https://registry.npmjs.org/
+ @username/hello-world@1.0.0
Test your package
Once published, you can access your package page by visiting: https://www.npmjs.com/package/@username/hello-world.
And you can test it by installing it within a test project using the npm install
command:
$ npm install --save @username/hello-world
Importing it within a JavaScript file:
const helloWorld = require('@username/hello-world');
helloWorld();
Running the script using the node
utility:
$ node index.js
Note that you should prefix the package name with your user or organization name only if the package is scoped.