May 14, 2023
Automatically Restart Your Node.js Application on File Change with Nodemon
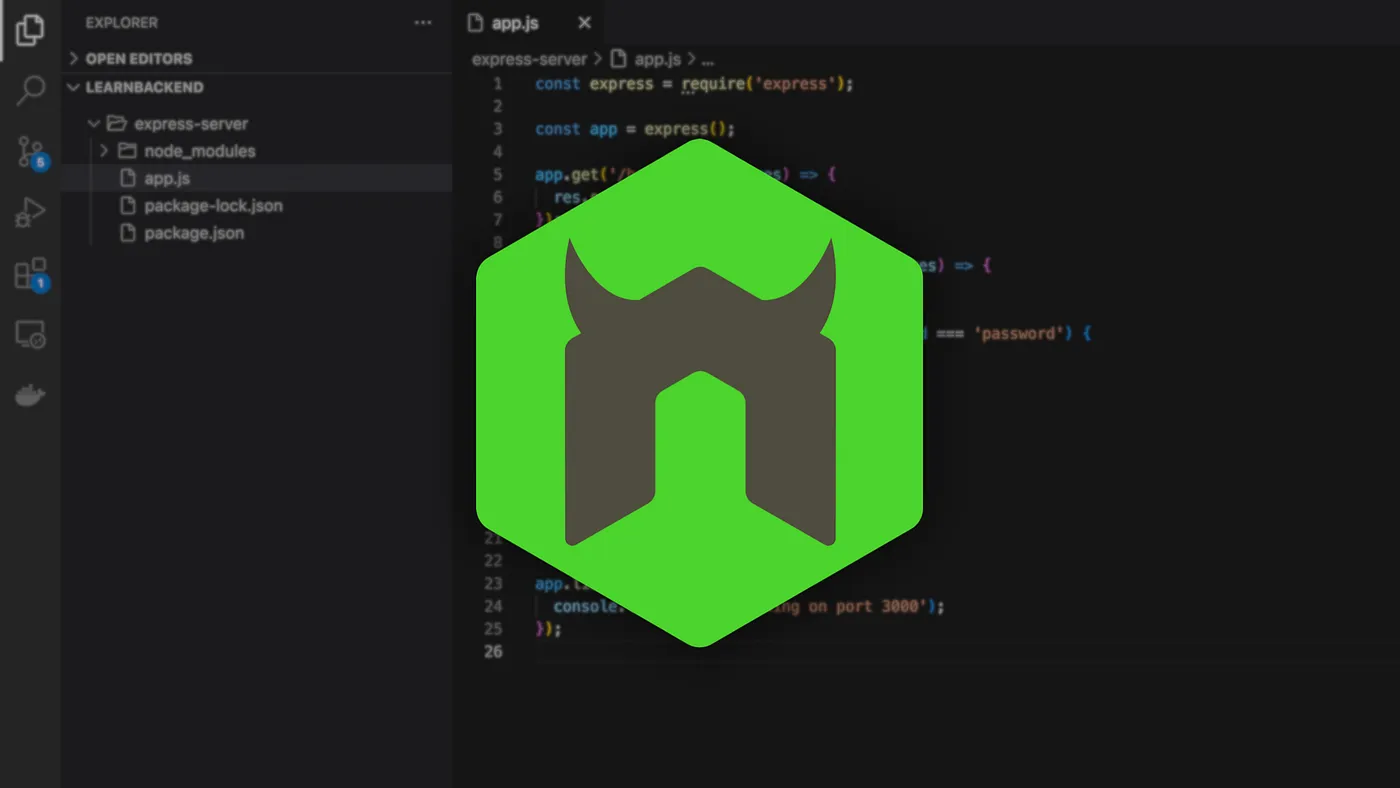
When developing with Node.js, we need to manually kill and restart our application every time we update the code in order for the changes to be applied.
In this article, you'll learn how to automate this process using a popular Node.js package named nodemon
.
Installing and using Nodemon
nodemon
is a command-line tool that watches the files in your project and automatically restarts your Node.js application whenever changes are detected.
Global
To install nodemon
as a global package, you can use the following command:
$ npm install -g nodemon
And then run any of your Node.js applications using the following command:
$ nodemon app.js
Local
To install nodemon
as a local package within a specific project, you can use the following command:
$ npm install --save-dev nodemon
Which will download and install it in the node_modules
directory, and save it in the devDependencies
of the package.json
file.
You can then add the following script to the package.json
file:
{
"scripts": {
"start": "nodemon app.js"
}
}
And run your application using the following command:
$ npm run start
Running a simple web server
Let's illustrate this using the following Express-powered web server that runs on the port 3000.
// File: app.js
const express = require('express');
const app = express();
const port = 3000;
app.listen(port, () => {
console.log(`Server running on port ${port}`);
});
When starting this application, nodemon will output the following logs in the terminal window, indicating which files are being watched.
$ nodemon app.js
[nodemon] 2.0.22
[nodemon] to restart at any time, enter `rs`
[nodemon] watching path(s): *.*
[nodemon] watching extensions: js,mjs,json
[nodemon] starting `node app.js`
Server running on port 3000
If you now change the port variable to 4000 instead.
// File: app.js
// ...
const port = 4000;
// ...
You should see the following log indicating that nodemon picked up the changes made and restarted the application.
[nodemon] restarting due to changes...
[nodemon] starting `node app.js`
Server running on port 4000
Watching and ignoring specific files
By default, nodemon will watch all files with the .js
, .mjs
, .coffee
, .litcoffee
, and .json
extensions located in the current directory and sub-directories.
Watching files
If you want to watch specific files, such as .env
environment files or directories (including sub-directories), you can use the --watch
flag combined with a file path or a globbing expression as follows:
$ nodemon --watch config/.env.development --watch utils app.js
Ignoring files
On the other hand, if you want to explicitly ignore certain files or directories, such as configuration or tests, you can use the --ignore
flag with a file path or a globbing expression as follows:
$ nodemon --ignore tests --ignore '*.json' app.js
Note that in both cases, globbing expressions must be quoted.
Useful option flags
Here is an overview of certain option flags you might find useful.
Delaying the application restart
By default, nodemon
will wait 1 second before restarting your application. If you want to change this parameter, you can use the --delay
flag as follows:
$ nodemon --delay 3 app.js
Note that this value can also be expressed in milliseconds using the 2.5
or 2500ms
syntax.
Watching specific extensions
If you want to change the list of file extensions watched by nodemon
, for example when using TypeScript, you can use the --ext
flag as follows:
$ nodemon --ext js,ts app.js
Using a configuration file
Instead of overpacking the nodemon
command with too many arguments, you can use a configuration file which takes any of the available option flags as JSON key values.
The nodemon.json file
The first option consists in creating a nodemon.json
file at the root of your project that contains your configuration as follows:
// File: nodemon.json
{
"delay": 3,
"watch": [
"config/.env.development",
"utils"
],
"ignore": [
"*.json",
"tests"
],
"ext": "ts"
}
The package.json file
On the other, if you want to keep all the configuration in the same place, you can specify all these values in the package.json
file under the nodemonConfig
property:
// File: package.json
{
"nodemonConfig": {
"delay": 3,
"watch": [
"config/.env.development",
"utils"
],
"ignore": [
"*.json",
"tests"
],
"ext": "ts"
}
}